Program Arcade Games
With Python And PygameChapter 1: Create a Custom Calculator
(Hi! If you don't already have a machine with Python and Pygame installed, then hop back to the “foreward” section to download and install them so you can get started.)
1.1 Introduction
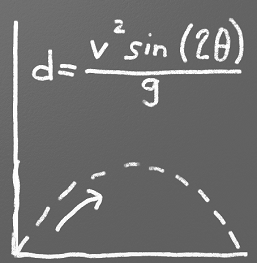
One of the simplest things that can be done with Python is to use it as a fancy calculator. Wait, a calculator isn't a game. Why are we talking about calculators? Boring....
Hey, to calculate objects dropping, bullets flying, and high scores, we need calculations. Plus, any true geek will consider a calculator a toy rather than a torture device! Let's start our game education with calculators. Don't worry, we'll start graphics by Chapter 5.
A simple calculator program can be used to ask the user for information and then calculate boring things like mortgage payments, or more exciting things like the trajectory of mud balls as they are flung through the air.
Figure 1.1 shows an example program that calculates kinetic energy, something we might need to do as part of a game physics engine.
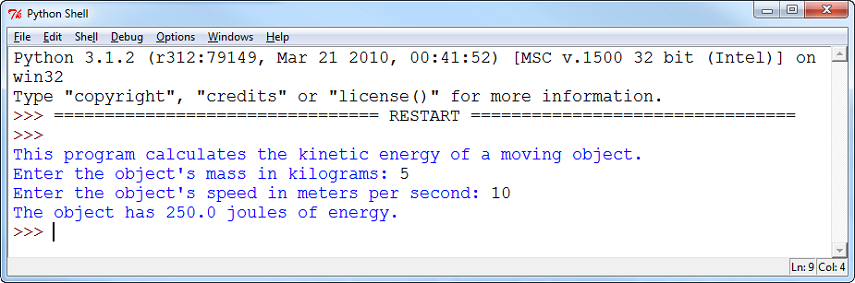
The best thing about doing this as a program is the ability to hide the complexities of an equation. All the user needs to do is supply the information and he or she can get the result in an easy-to-understand format. Any similar custom calculator could run on a smart phone, allowing a person to easily perform the calculation on the go.
1.2 Printing
1.2.1 Printing Text
How does a program print something to the screen? The code is simple. Just one line is required:
print("Hello World.")
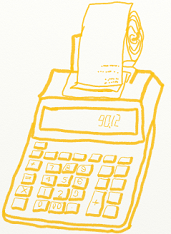
This program prints out “Hello World” to the screen. Go ahead and enter it into IDLE prompt and see how it works. Try printing other words and phrases as well. The computer will happily print out just about anything you like, true or not.
What does the “Hello World” program look like in other computer programming
languages? Check out Wikibooks.
They keep a nice set of “Hello World” programs written
in many different computer programming languages:
https://en.wikibooks.org/wiki/Computer_Programming/Hello_world
It is interesting to see how many different computer languages there are. You can get an idea how complex a language is by how easy the “Hello World” program is.
Remember, the command for printing in Python is easy. Just use print. After the print command are a set of parentheses ( ). Inside these parentheses is what should be printed to the screen. Using parentheses to pass information to a function is standard practice in math, and computer languages.
Math students learn to use parentheses evaluating expressions like $sin(\theta)=cos(\frac{\pi}{2}-\theta)$. $sin$ and $cos$ are functions. Data passed to these functions is inside the parenthesis. What is different in our case is that the information being passed is text.
Notice that there are double quotes around the text to be printed. If a print statement has quotes around text, the computer will print it out just as it is written. For example, this program will print 2+3:
print("2 + 3")
1.2.2 Printing Results of Expressions
This next program does not have quotes around $2+3$, and the computer will evaluate it as a mathematical expression. It will print 5 rather than 2+3.
print(2 + 3)
The code below will generate an error because the computer will try to evaluate “Hello World” as a mathematical expression, and that doesn't work at all:
print(Hello World)
The code above will print out an error SyntaxError: invalid syntax which is computer-speak for not knowing what “Hello” and “World” mean.
Also, please keep in mind that this is a single-quote: ' and this is a double-quote: " If I ask for a double-quote, it is a common mistake to write "" which is really a double double-quote.
1.2.3 Printing Multiple Items
A print statement can output multiple things at once, each item separated by a comma. For example this code will print out Your new score is 1040
print("Your new score is", 1030 + 10)
The next line of code will print out Your new score is 1030+10. The numbers are not added together because they are inside the quotes. Anything inside quotes, the computer treats as text. Anything outside the computer thinks is a mathematical statement or computer code.
print("Your new score is", "1030 + 10")
This next code example doesn't work at all. This is because there is no comma separating the text between the quotes, and the 1030+10. At first, it may appear that there is a comma, but the comma is inside the quotes. The comma that separates the terms to be printed must be outside the quotes. If the programmer wants a comma to be printed, then it must be inside the quotes:
print("Your new score is," 1030 + 10)
This next example does work, because there is a comma separating the terms. It prints:
Your new score is, 1040
Note that only one comma prints out. Commas outside the quotes separate terms, commas inside
the quotes are printed. The first comma is printed, the second is used to separate terms.
print("Your new score is,", 1030 + 10)
1.3 Escape Codes
If quotes are used to tell the computer the start and end of the string of text you wish to print, how does a program print out a set of double quotes? For example:
print("I want to print a double quote " for some reason.")
This code doesn't work. The computer looks at the quote in the middle of the string and thinks that is the end of the text. Then it has no idea what to do with the commands for some reason and the quote and the end of the string confuses the computer even further.
It is necessary to tell the computer that we want to treat that middle double quote as text, not as a quote ending the string. This is easy, just prepend a backslash in front of quotes to tell the computer it is part of a string, not a character that terminates a string. For example:
print("I want to print a double quote \" for some reason.")
This combination of the two characters \" is called an escape code. Almost every language has them. Because the backslash is used as part of an escape code, the backslash itself must be escaped. For example, this code does not work correctly:
print("The file is stored in C:\new folder")
Why? Because \n is an escape code. To print the backslash it is necessary to escape it like so:
print("The file is stored in C:\\new folder")
There are a few other important escape codes to know. Here is a table of the important escape codes:
Escape code | Description |
---|---|
\' | Single Quote |
\" | Double Quote |
\t | Tab |
\r | CR: Carriage Return (move to the left) |
\n | LF: Linefeed (move down) |
What is a “Carriage Return” and a “Linefeed”? Try this example:
print("This\nis\nmy\nsample.")
The output from this command is:
This is my sample.
The \n is a linefeed. It moves “cursor” where the computer will print text down one line. The computer stores all text in one big long line. It knows to display the text on different lines because of the placement of \n characters.
To make matters more complex, different operating systems have different standards on what makes a line ending.
Escape codes | Description |
---|---|
\r\n | CR+LF: Microsoft Windows |
\n | LF: UNIX based systems, and newer Macs. |
\r | CR: Older Mac based systems |
Usually your text editor will take care of this for you. Microsoft Notepad doesn't though, and UNIX files opened in notepad look terrible because the line endings don't show up at all, or show up as black boxes. Every programmer should have a good text editor installed on their computer. I recommend Sublime or Notepad++.
1.4 Comments
Sometimes code needs some extra explanation to the person reading it. To do this, we add “comments” to the code. The comments are meant for the human reading the code, and not for the computer.
There are two ways to create a comment. The first is to use the # symbol. The computer will ignore any text in a Python program that occurs after the #. For example:
# This is a comment, it begins with a # sign # and the computer will ignore it. print("This is not a comment, the computer will") print("run this and print it out.")
If a program has the # sign between quotes it is not treated as a comment. A programmer can disable a line of code by putting a # sign in front of it. It is also possible to put a comment in at the end of a line.
print("A # sign between quotes is not a comment.") # print("This is a comment, even if it is computer code.") print("Hi") # This is an end-of-line comment
It is possible to comment out multiple lines of code using three single quotes in a row to delimit the comments.
print("Hi") ''' This is a multi line comment. Nothing Will run in between these quotes. print("There") ''' print("Done")
Most professional Python programmers will only use this type of multi-line comment for something called docstrings. Docstrings allow documentation to be written along side the code and later be automatically pulled out into printed documentation, websites, and Integrated Development Environments (IDEs). For general comments, the # tag works best.
Even if you are going to be the only one reading the code that you write, comments can help save time. Adding a comment that says “Handle alien bombs” will allow you to quickly remember what that section of code does without having to read and decipher it.
1.5 Assignment Operators
How do we store the score in our game? Or keep track of the health of the enemy? What we need to do this is the assignment operator.
An operator is a symbol like + or -. An assignment operator is the = symbol. It stores a value into a variable to be used later on. The code below will assign 10 to the variable x, and then print the value stored in x.
Look at the example below. Click on the “Step” button to see how the code operates.
# Create a variable x # Store the value 10 into it. x = 10 # This prints the value stored in x. print(x) # This prints the letter x, but not the value in x print("x") # This prints "x= 10" print("x=", x) |
Variables:
x= Output:
10 x x= 10 |
Note: The listing above also demonstrates the difference between printing
an x inside quotes and an x outside quotes.
If an x is inside quotation
marks, then the computer prints x. If an x is outside
the quotation marks then the computer will print the value of x.
Getting confused on the “inside or outside of quotes” question
is very common for those learning to program.
An assignment statement (a line of code using the = operator) is different than the algebraic equality your learned about in math. Do not think of them as the same. On the left side of an assignment operator must be exactly one variable. Nothing else may be there.
On the right of the equals sign/assignment operator is an expression. An expression is anything that evaluates to a value. Examine the code below.
x = x + 1
The code above obviously can't be an algebraic equality. But it is valid to the computer because it is an assignment statement. Mathematical equations are different than assignment statements even if they have variables, numbers, and an equals sign.
The code in the above statement takes the current value of x, adds one to it, and stores the result back into x.
Expanding our example, the statement below will print the number 6.
x = 5 x = x + 1 print(x)
Statements are run sequentially. The computer does not “look ahead.” In the code below, the computer will print out 5 on line 2, and then line 4 will print out a 6. This is because on line 2, the code to add one to x has not been run yet.
x = 5 print(x) # Prints 5 x = x + 1 print(x) # Prints 6
The next statement is valid and will run, but it is pointless. The computer will add one to x, but the result is never stored or printed.
x + 1
The code below will print 5 rather than 6 because the programmer forgot to store the result of x + 1 back into the variable x.
x = 5 x + 1 print(x)
The statement below is not valid because on the left of the equals sign is more than just a variable:
x + 1 = x
Python has other types of assignment operators. They allows a programmer to modify a variable easily. For example:
x += 1
The above statement is equivalent to writing the code below:
x = x + 1
There are also assignment operators for addition, subtraction, multiplication and division.
1.6 Variables
Variables should start with a lower case letter. Variables can start with an upper case letter or an underscore, but those are special cases and should not be done on a normal basis. After the first lower case letter, the variable may include uppercase and lowercase letters, along with numbers and underscores. Variables may not include spaces.
Variables are case sensitive. This can be confusing if a programmer is not expecting it. In the code below, the output will be 6 rather than 5 because there are two different variables, x and X.
x = 6 X = 5 print(x)
The official style guide for Python (yes, programmers really wrote a book on style) says that multi-word variable names in Python should be separated by underscores. For example, use hair_style and not hairStyle. Personally, if you are one of my students, I don't care about this rule too much because the next language we introduce, Java, has the exact opposite style rule. I used to try teaching Java style rules while in this class, but then I started getting hate-mail from Python lovers. These people came by my website and were shocked, shocked I tell you, about my poor style.
Joan Rivers has nothing on these people, so I gave up and try to use proper style guides now.
Here are some example variable names that are ok, and not ok to use:
Legal variable names | Illegal variable names | Legal, but not proper |
---|---|---|
first_name | first name | FirstName |
distance | 9ds | firstName |
ds9 | %correct | X |
All upper-case variable names like MAX_SPEED are allowed only in circumstances where the variable's value should never change. A variable that isn't variable is called a constant.
1.7 Operators
For more complex mathematical operations, common mathematical operators are available. Along with some not-so-common ones:
operator | operation | example equation | example code |
---|---|---|---|
+ | addition | $3 + 2$ | a = 3 + 2 |
- | subtraction | $3 - 2$ | a = 3 - 2 |
* | multiplication | $3 \cdot 2$ | a = 3 * 2 |
/ | division | $\frac{10}{2}$ | a = 10 / 2 |
// | floor division | N/A | a = 10 // 3 |
** | power | $2^3$ | a = 2 ** 3 |
% | modulus | N/A | a = 8 % 3 |
“Floor division” will always round the answer down to the nearest integer. For example, 11//2 will be 5, not 5.5, and 99//100 will equal 0.
Multiplication by juxtaposition does not work in Python. The following two lines of code will not work:
# These do not work x = 5y x = 5(3/2)
It is necessary to use the multiplication operator to get these lines of code to work:
# These do work x = 5 * y x = 5 * (3 / 2)
1.7.1 Operator Spacing
There can be any number of spaces before and after an operator, and the computer will understand it just fine. For example each of these three lines are equivalent:
x=5*(3/2) x = 5 * (3 / 2) x =5 *( 3/ 2)
The official style guide for Python says that there should be a space before and after each operator. (You've been dying to know, right? Ok, the official style guide for python code is called: PEP-8. Look it up for more excitement.) Of the three lines of code above, the most “stylish” one would be line 2.
1.8 Order of Operations
Python will evaluate expressions using the same order of operations that are expected in standard mathematical expressions. For example this equation does not correctly calculate the average:
average = 90 + 86 + 71 + 100 + 98 / 5
The first operation done is 98/5. The computer calculates:
$90+86+71+100+\frac{98}{5}$
rather than the desired:
$\dfrac{90+86+71+100+98}{5}$
By using parentheses this problem can be fixed:
average = (90 + 86 + 71 + 100 + 98) / 5
1.9 Trig Functions
Trigonometric functions are used to calculate sine and cosine in equations. By default, Python does not know how to calculate sine and cosine, but it can once the proper library has been imported. Units are in radians.
# Import the math library # This line is done only once, and at the very top # of the program. from math import * # Calculate x using sine and cosine x = sin(0) + cos(0)
1.10 Custom Equation Calculators
A program can use Python to calculate the mileage of a car that drove 294 miles on 10.5 gallons of gas.
m = 294 / 10.5 print(m)
This program can be improved by using variables. This allows the values to easily be changed in the code without modifying the equation.
m = 294 g = 10.5 m2 = m / g # This uses variables instead print(m2)
By itself, this program is actually difficult to understand. The variables m and g don't mean a lot without some context. The program can be made easier to understand by using appropriately named variables:
miles_driven = 294 gallons_used = 10.5 mpg = miles_driven / gallons_used print(mpg)
Now, even a non-programmer can probably look at the program and have a good idea of what it does. Another example of good versus bad variable naming:
# Hard to understand ir = 0.12 b = 12123.34 i = ir * b # Easy to understand interest_rate = 0.12 account_balance = 12123.34 interest_amount = interest_rate * account_balance
In the IDLE editor it is possible to edit a prior line without retyping it. Do this by moving the cursor to that line and hitting the “enter” key. It will be copied to the current line.
Entering Python code at the >>> prompt is slow and can only be done one line at a time. It is also not possible to save the code so that another person can run it. Thankfully, there is an even better way to enter Python code.
Python code can be entered using a script. A script is a series of lines of Python
code that will be executed all at once. To create a script, open up a new window as
shown in Figure 1.2.
You may wish to use a different program to create your script, like the Wing IDE or PyCharm. These programs are easier and more powerful than the IDLE program that comes with Python.)
Enter the Python program for calculating gas mileage, and then save the file.
Save the file to a flash drive, network drive, or some other location of your
choice. Python programs should always end with .py.
See Figure 1.3.
Run the program typed in by clicking on the “Run” menu and selecting “Run Module”. Try updating the program to different values for miles driven and gallons used.
From this point forward, almost all code entered should be in a script/module. Do not type your program out on the IDLE >>> prompt. Code typed here is not saved. If this happens, it will be necessary to start over. This is a very common mistake for new programmers.
This program would be even more useful if it would interact with the user and ask the user for the miles driven and gallons used. This can be done with the input statement. See the code below:
# This code almost works miles_driven = input("Enter miles driven:") gallons_used = input("Enter gallons used:") mpg = miles_driven / gallons_used print("Miles per gallon:", mpg)
Running this program will ask the user for miles and gallons, but it generates a strange
error as shown in Figure 1.4.
The reason for this error can be demonstrated by changing the program a bit:
miles_driven = input("Enter miles driven:") gallons_used = input("Enter gallons used:") x = miles_driven + gallons_used print("Sum of m + g:", x)
Running the program above results in the output shown in
Figure 1.5.
The program doesn't add the two numbers together, it just puts one right after the other. This is because the program does not know the user will be entering numbers. The user might enter “Bob” and “Mary”, and adding those two variables together would be “BobMary”; which would make more sense.
To tell the computer these are numbers, it is necessary to surround the input function with an int( ) or a float( ). Use the former for integers, and the latter for floating point numbers.
The final working program:
# Sample Python/Pygame Programs # Simpson College Computer Science # http://programarcadegames.com/ # http://simpson.edu/computer-science/ # Explanation video: http://youtu.be/JK5ht5_m6Mk # Calculate Miles Per Gallon print("This program calculates mpg.") # Get miles driven from the user miles_driven = input("Enter miles driven:") # Convert text entered to a # floating point number miles_driven = float(miles_driven) # Get gallons used from the user gallons_used = input("Enter gallons used:") # Convert text entered to a # floating point number gallons_used = float(gallons_used) # Calculate and print the answer mpg = miles_driven / gallons_used print("Miles per gallon:", mpg) |
Variables:
milesDriven= gallonsUsed= mpg= Output:
This program calculates mpg. Enter miles driven: 288 Enter gallons used: 15 Miles per gallon: 19.2 |
And another example, calculating the kinetic energy of an object:
# Sample Python/Pygame Programs # Simpson College Computer Science # http://programarcadegames.com/ # http://simpson.edu/computer-science/ # Calculate Kinetic Energy print("This program calculates the kinetic energy of a moving object.") m_string = input("Enter the object's mass in kilograms: ") m = float(m_string) v_string = input("Enter the object's speed in meters per second: ") v = float(v_string) e = 0.5 * m * v * v print("The object has " + str(e) + " joules of energy.")
To shorten a program, it is possible to nest the input statement into the float statement. For example these lines of code:
milesDriven = input("Enter miles driven:") milesDriven = float(milesDriven)
Perform the same as this line:
milesDriven = float(input("Enter miles driven:"))
In this case, the output of the input function is directly fed into the float function. Either one works, and it is a matter of programmer's preference which to choose. It is important, however, to be able to understand both forms.
1.11 Review
1.11.1 Multiple Choice Quiz
Click here for a multiple-choice quiz.
1.11.2 Short Answer Worksheet
Click here for the chapter worksheet.
1.11.3 Lab
Click here for the chapter lab.
You are not logged in. Log in here and track your progress.
English version by Paul Vincent Craven
Spanish version by Antonio Rodríguez Verdugo
Russian version by Vladimir Slav
Turkish version by Güray Yildirim
Portuguese version by Armando Marques Sobrinho and Tati Carvalho
Dutch version by Frank Waegeman
Hungarian version by Nagy Attila
Finnish version by Jouko Järvenpää
French version by Franco Rossi
Korean version by Kim Zeung-Il
Chinese version by Kai Lin