Program Arcade Games
With Python And PygameLab 1: Custom Calculators
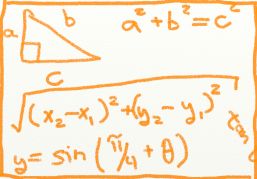
In this lab we'll create three custom calculator programs. To help create these labs check the code in Chapter 1. In particular, the example program at the end of that chapter provides a good template for the code needed in this lab.
Make sure you can write out simple programs like what is assigned in this lab. Be able to do it from memory, and on paper. These programs follow a very common pattern in computing:
- Take in data
- Perform calculations
- Output data
Programs take in data from sources like databases, 3D models, game controllers, keyboards, and the Internet. They perform calculations and output the result. Sometimes we even do this in a loop thousands of times a second.
It is a good idea to do the calculations separate from the output of the data. While it is possible to do the calculation inside the print statement, it is better to do the calculation, store it in a variable, and then output it later. This way calculations and output aren't mixed together.
When writing programs it is a good idea to use blank lines to separate logical groupings of code. For example, place a blank line between the input statements, the calculation, and the output statement. Also, add comments to your program labeling these sections.
For this lab you will create three short programs. If you are using a version control system, remember to commit and push your changes to the server. Click the “Send the lab for grading” button when you are done.
1.1 Part A
Create a program that asks the user for a temperature in Fahrenheit, and then prints the temperature in Celsius. Search the Internet for the correct calculation. Look at Chapter 1 for the miles-per-gallon example to get an idea of what should be done.
Sample run:
Enter temperature in Fahrenheit: 32 The temperature in Celsius: 0.0
Sample run:
Enter temperature in Fahrenheit: 72 The temperature in Celsius: 22.2222222222
The numbers from this program won't be formatted nicely. That is ok. But if it bothers you, look ahead to Chapter 20 and see how to make your output look great!
Make sure to spell "Celsius" and "Fahrenheit" correctly. When printing an input prompt, use proper grammar and capitalization. Don't lose points for English.
1.2 Part B
Create a new program that will ask the user for the information needed
to find the area of a trapezoid, and then print the area. The formula for
the area of a trapezoid is:
$A=\dfrac{1}{2}(x_1+x_2)h$
Sample run:
Area of a trapezoid Enter the height of the trapezoid: 5 Enter the length of the bottom base: 10 Enter the length of the top base: 7 The area is: 42.5
1.3 Part C
Create your own original problem and have the user plug in the variables. If you are not in the mood for anything original, choose an equation from this list:
Area of a circle | $A=\pi r^2$ |
Area of an ellipse | $A=\pi r_1 r_2$ |
Area of an equilateral triangle | $A=\dfrac{h^2 \sqrt{3}}{3}$ |
Volume of a cone | $V=\dfrac{\pi r^2h}{3}$ |
Volume of a sphere | $V=\dfrac{4\pi r^3}{3}$ |
Area of an arbitrary triangle | $A=\dfrac{1}{2}ab\sin C$ |
When done, check to make certain your variable names begin with a lower case letter, and that you are using blank lines between logical groupings of the code. (Between input, calculations, and output in this case.)
Then, turn in the assignment according to your teacher's instructions. If you are using a version control system, make sure to commit the files, and push them to the server.
You are not logged in. Log in here and track your progress.
English version by Paul Vincent Craven
Spanish version by Antonio Rodríguez Verdugo
Russian version by Vladimir Slav
Turkish version by Güray Yildirim
Portuguese version by Armando Marques Sobrinho and Tati Carvalho
Dutch version by Frank Waegeman
Hungarian version by Nagy Attila
Finnish version by Jouko Järvenpää
French version by Franco Rossi
Korean version by Kim Zeung-Il
Chinese version by Kai Lin